hybridsjs/hybrids
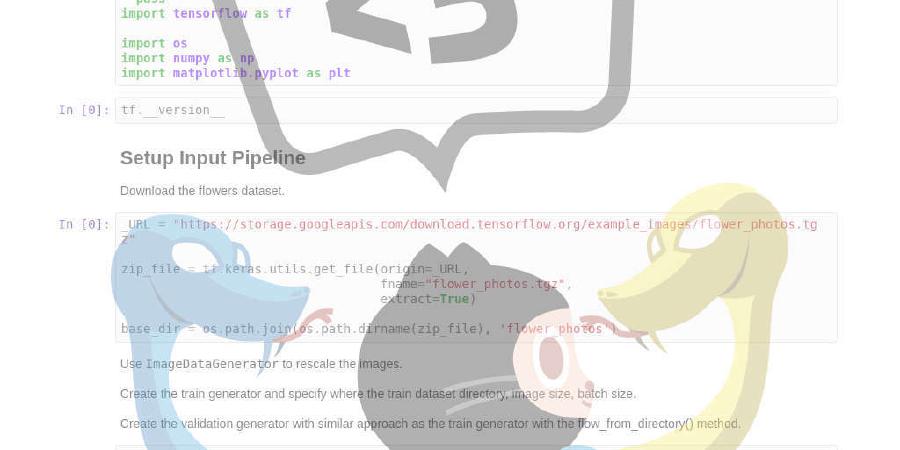
The simplest way to create web components from plain objects and pure functions!
repo name | hybridsjs/hybrids |
repo link | https://github.com/hybridsjs/hybrids |
homepage | https://hybrids.js.org |
language | JavaScript |
size (curr.) | 2035 kB |
stars (curr.) | 1753 |
created | 2016-12-06 |
license | MIT License |
🏅One of the four nominated projects to the “Breakthrough of the year” category of Open Source Award in 2019
Hybrids is a UI library for creating web components with strong declarative and functional approach based on plain objects and pure functions.
- The simplest definition — just plain objects and pure functions - no
class
andthis
syntax - No global lifecycle — independent properties with own simplified lifecycle methods
- Composition over inheritance — easy re-use, merge or split property definitions
- Super fast recalculation — built-in smart cache and change detection mechanisms
- Templates without external tooling — template engine based on tagged template literals
- Developer tools included — Hot module replacement support for a fast and pleasant development
Getting Started
Install npm package:
Then, import the required features and define a custom element:
import { html, define } from 'hybrids';
export function increaseCount(host) {
host.count += 1;
}
export const SimpleCounter = {
count: 0,
render: ({ count }) => html`
<button onclick="${increaseCount}">
Count: ${count}
</button>
`,
};
define('simple-counter', SimpleCounter);
👆 Click and play on ⚡StackBlitz
Finally, use your custom element in HTML:
<simple-counter count="10"></simple-counter>
ES Modules
If you target modern browsers and do not want to use external tooling (like webpack or parcel), you can use ES modules:
<script type="module">
// We can use "/src" here - browsers, which support modules also support ES2015
import { html, define } from 'https://unpkg.com/hybrids@[PUT_VERSION_HERE:x.x.x]/src';
...
</script>
Please take to account, that it does not provide code minification and loads all required files in separate requests.
Built Version
For older browsers support you can use the built version (with window.hybrids
global namespace):
<script src="https://unpkg.com/hybrids@[PUT_VERSION_HERE:x.x.x]/dist/hybrids.js"></script>
<script>
var define = window.hybrids.define;
var html = window.hybrids.html;
...
</script>
Hot Module Replacement
HMR works out of the box, but your bundler setup may require indication that your entry point supports it. For webpack
and parcel
add the following code to your entry point:
// Enable HMR for development
if (process.env.NODE_ENV !== 'production') module.hot.accept();
If your entry point imports files that do not support HMR, you can place the above snippet in a module where you define a custom element. (where define
method is used).
Overview
There are some common patterns among JavaScript UI libraries like class syntax, a complex lifecycle or stateful architecture. What can we say about them?
Classes can be confusing, especially about how to use this
or super()
calls. They are also hard to compose. Multiple lifecycle callbacks have to be studied to understand very well. A stateful approach can open doors for difficult to maintain, imperative code. Is there any way out from all of those challenges?
After all, the class syntax in JavaScript is only sugar on top of the constructors and prototypes. Because of that, we can switch the component structure to a map of properties applied to the prototype of the custom element class constructor. Lifecycle callbacks can be minimized with smart change detection and cache mechanism. Moreover, they can be implemented independently in the property scope rather than globally in the component definition.
With all of that, the code may become simple to understand, and the code is written in a declarative way. Not yet sold? You can read more in the Core Concepts section of the project documentation.
Documentation
The hybrids documentation is available at hybrids.js.org or in the docs path of the repository:
Articles
Core Concepts Series
- From classes to plain objects and pure functions
- Say goodbye to lifecycle methods, and focus on productive code
- Chasing the best performance of rendering the DOM by hybrids library
- Three unique features of the hybrids template engine that you must know
Videos
- Taste the Future with Functional Web Components (EN, ConFrontJS Conference)
- Hybrids - Web Components with Simple and Functional API (PL, WarsawJS Meetup #46)
Live Examples
- <simple-counter> - a button with counter controlled by own state
- <redux-counter> - Redux library for state management
- <react-counter> - render factory and React library for rendering in shadow DOM
- <lit-counter> - render factory and lit-html for rendering in shadow DOM
- <app-todos> - todo list using parent factory for state management
- <tab-group> - switching tabs using children factory
- <async-user> - async data in the template
Browser Support
The library requires some of the ES2015 APIs and Shadow DOM, Custom Elements, and Template specifications. You can use hybrids
in all evergreen browsers and IE11 including a list of required polyfills and shims. The easiest way is to add a bundle from @webcomponents/webcomponentsjs
package on top of your project:
import '@webcomponents/webcomponentsjs/webcomponents-bundle.js';
import { define, ... } from 'hybrids';
...
The polyfill package provides two modes in which you can use it (webcomponents-bundle.js
and webcomponents-loader.js
). Read more in the How to use section of the documentation.
Web components shims have some limitations. Especially, webcomponents/shadycss
approximates CSS scoping and CSS custom properties inheritance. Read more on the known issues and custom properties shim limitations pages.
License
hybrids
is released under the MIT License.