MindorksOpenSource/android-interview-questions
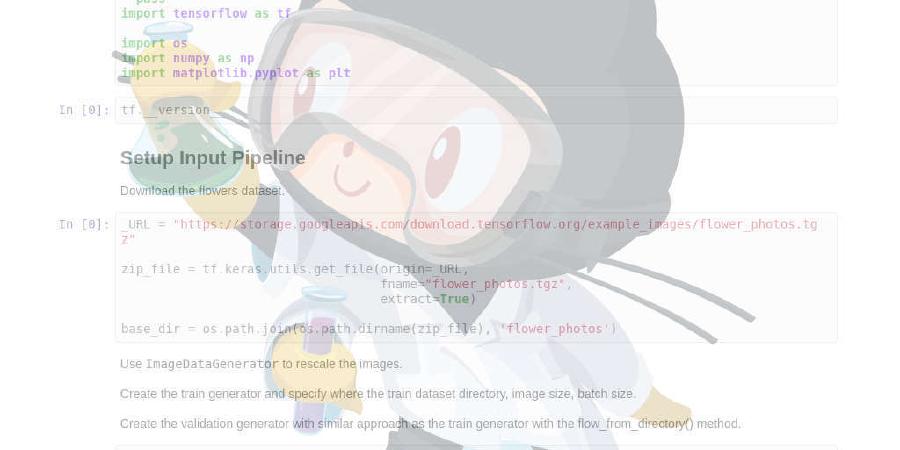
Your Cheat Sheet For Android Interview - Android Interview Questions
repo name | MindorksOpenSource/android-interview-questions |
repo link | https://github.com/MindorksOpenSource/android-interview-questions |
homepage | https://mindorks.com |
language | Java |
size (curr.) | 1226 kB |
stars (curr.) | 5586 |
created | 2017-07-09 |
license | Apache License 2.0 |
Android Interview Questions
Android Interview Questions - Your Cheat Sheet For Android Interview
Prepared and maintained by Amit Shekhar who is having experience of taking interviews of many Android developers and cracking interviews of top companies.
Join and learn here
Contents
Data Structures And Algorithms
The level of questions asked on the topic of Data Structures And Algorithms totally depends on the company for which you are applying.
Check Whiteboard Interview Series - Data Structures and Algorithms on Youtube
Tech Interview Preparation Kit
Android Developer should know these Data Structures for Next Interview
-
Array
- An Array consists of a group of elements of the same data type. It is stored contiguously in memory and by using its' index, you can find the underlying data. Arrays can be one dimensional and multi-dimensional. One dimensional array is the simplest data structure, and also most commonly used. It is worth noting that in Java language multi-dimensional arrays are implemented as arrays of arrays. For example,
int[10][5]
is actually one array with its' cells pointing to ten 5-element arrays.Algorithm Average Worst Case Space Θ(n) O(n) Search Θ(n) O(n) Insert Θ(n) O(n) Delete Θ(n) O(n)
- An Array consists of a group of elements of the same data type. It is stored contiguously in memory and by using its' index, you can find the underlying data. Arrays can be one dimensional and multi-dimensional. One dimensional array is the simplest data structure, and also most commonly used. It is worth noting that in Java language multi-dimensional arrays are implemented as arrays of arrays. For example,
-
LinkedList
- A LinkedList, just like a tree and unlike an array, consists of a group of nodes which
together represent a sequence. Each node contains data and a pointer. The data in a node can be
anything, but the pointer is a reference to the next item in the LinkedList. A LinkedList
contains both a head and a tail. The “Head” is the first item in the LinkedList, while the “Tail” is
the last item. It is not a circular data structure, therefore the tail does not have its'
pointer pointing at the Head - the pointer is just
null
. The run time complexity for each of the base methods are as follows:Algorithm Average Worst Case Space Θ(n) O(n) Search Θ(n) O(n) Insert Θ(1) O(1) Delete Θ(1) O(1)
- A LinkedList, just like a tree and unlike an array, consists of a group of nodes which
together represent a sequence. Each node contains data and a pointer. The data in a node can be
anything, but the pointer is a reference to the next item in the LinkedList. A LinkedList
contains both a head and a tail. The “Head” is the first item in the LinkedList, while the “Tail” is
the last item. It is not a circular data structure, therefore the tail does not have its'
pointer pointing at the Head - the pointer is just
-
DoublyLinkedList
-
A DoublyLinkedList is based on a LinkedList, but there is two pointers in each node, “previous” pointer holds reference to the previous node and “next” pointer holds reference to the next node. It also has a Head node, head node’s next pointer references the first node in this DoublyLinkedList. The last node’s “next” reference points to
null
, but if last node’s next pointer points to the first node, such DoublyLinkedList is called “Circular DoublyLinkedList”. This data structure is very convenient if you need to be able to traverse stored elements in both directions.Algorithm Average Worst Case Space Θ(n) O(n) Search Θ(n) O(n) Insert Θ(1) O(1) Delete Θ(1) O(1)
-
-
Stack
- A Stack is a basic data structure with a “Last-in-First-out” (LIFO) semantics. This means that
the last item that was added to the stack is the first item that comes out of the stack. A
Stack is like a stack of books in that in order to get to the first book that was added in the stack
(the bottom book), all of the books that were added after need to be removed first. Adding to a
Stack is called “Push”, removing from a stack is called “Pop”, and getting the last item
inserted into the stack without removing it is called “Top”. The most common way to implement a
stack is by using a LinkedList, but there is also StackArray (implemented with an array)
which does not replace null entries, and there is also a Vector implementation that does
replace
null
entries. Wikipedia
- A Stack is a basic data structure with a “Last-in-First-out” (LIFO) semantics. This means that
the last item that was added to the stack is the first item that comes out of the stack. A
Stack is like a stack of books in that in order to get to the first book that was added in the stack
(the bottom book), all of the books that were added after need to be removed first. Adding to a
Stack is called “Push”, removing from a stack is called “Pop”, and getting the last item
inserted into the stack without removing it is called “Top”. The most common way to implement a
stack is by using a LinkedList, but there is also StackArray (implemented with an array)
which does not replace null entries, and there is also a Vector implementation that does
replace
-
Queue
-
PriorityQueue
-
Binary Tree Wikipedia
-
Binary Search Tree
-
Hash Table or Hash Map
-
Sorting Algorithms Wikipedia
- Using the most efficient sorting algorithm (and correct data structures that implement it) is vital for any program, because data manipulation can be one of the most significant bottlenecks in case of performance and the main purpose of spending time, determining the best algorithm for the job, is to drastically improve said performance. The efficiency of an algorithm is measured in its' “Big O” (StackOverflow) score. Really good algorithms perform important actions in O(n log n) or even O(log n) time and some of them can even perform certain actions in O(1) time (HashTable insertion, for example). But there is always a trade-off - if some algorithm is really good at adding a new element to a data structure, it is, most certainly, much worse at data access than some other algorithm. If you are proficient with math, you may notice that “Big O” notation has many similarities with “limits”, and you would be right - it measures best, worst and average performances of an algorithm in question, by looking at its' function limit. It should be noted that, when we are speaking about O(1) - constant time - we are not saying that this algorithm performs an action in one operation, rather that it can perform this action with the same number of operations (roughly), regrardless of the amount of elements it has to take into account. Thankfully, a lot of “Big O” scores have been already calculated, so you don’t have to guess, which algorithm or data structure will perform better in your project. “Big O” cheat sheet
- Bubble sort Wikipedia
- Bubble sort is one of the simplest sorting algorithms. It just compares neighbouring elements and if the one that precedes the other is smaller - it changes their places. So over one iteration over the data list, it is guaranteed that at least one element will be in its' correct place (the biggest/smallest one - depending on the direction of sorting). This is not a very efficient algorithm, as highly unordered arrays will require a lot of reordering (upto O(n^2)), but one of the advantages of this algorithm is its' space complexity - only two elements are compared at once and there is no need to allocate more memory, than those two will occupy.
- Selection sort Wikipedia
- Firstly, selection sort assumes that the first element of the array to be sorted is the smallest, but to confirm this, it iterates over all other elements to check, and if it finds one, it gets defined as the smallest one. When the data ends, the element, that is currently found to be the smallest, is put in the beginning of the array. This sorting algorithm is quite straightforward, but still not that efficient on larger data sets, because to assign just one element to its' place, it needs to go over all data.
- Insertion sort Wikipedia
- Insertion sort is another example of an algorithm, that is not that difficult to implement, but is also not that efficient. To do its' job, it “grows” sorted portion of data, by “inserting” new encountered elements into already (innerly) sorted part of the array, which consists of previously encountered elements. This means that in best case (data is already sorted) it can confirm that its' job is done in Ω(n) operations, while, if all encountered elements are not in their required order as many as O(n^2) operations may be needed.
- Merge sort Wikipedia
- This is a “divide and conquer” algorithm, meaning it recursively “divides” given array in to smaller parts (up to 1 element) and then sorts those parts, combining them with each other. This approach allows merge sort to achieve very high speed, while doubling required space, of course, but today memory space is more available than it was a couple of years ago, so this trade-off is considered acceptable.
- This is a “divide and conquer” algorithm, meaning it recursively “divides” given array in to smaller parts (up to 1 element) and then sorts those parts, combining them with each other. This approach allows merge sort to achieve very high speed, while doubling required space, of course, but today memory space is more available than it was a couple of years ago, so this trade-off is considered acceptable.
- Quicksort Wikipedia
- Quicksort is considered, well, quite quick. When implemented correctly, it can be a significant number of times faster than its' main competitors. This algorithm is also of “divide and conquer” family and its' first step is to choose a “pivot” element (choosing it randomly, statistically, minimizes the chance to get the worst performance), then by comparing elements to this pivot, moving it closer and closer to its' final place. During this process, the elements that are bigger are moved to the right side of it and smaller elements to the left. After this is done, quicksort repeats this process for subarrays on each side of placed pivot (does first step recursively), until the array is sorted. Time Complexity Space Complexity Best Average Worst Worst Ω(n log(n)) Θ(n log(n)) O(n^2) O(n)
- There are, of course, more sorting algorithms and their modifications. We strongly recommend all readers to familiarize themselves with a couple more, because knowing algorithms is very important quality of a candidate, applying for a job and it shows understanding of what is happening “under the hood”.
-
Dynamic Programming
-
Greedy Algorithm
-
String Manipulation
-
Pathfinding algorithms Wikipedia
- Dijkstra algorithm
- A* algorithm
- Breadth First Search
- Depth First Search
Core Java
OOP
-
Explain OOP Concepts.
- Object-Oriented Programming is a methodology of designing a program using classes, objects, inheritance, polymorphism, abstraction, and encapsulation.
-
Differences between abstract classes and interfaces? GitHub
- An abstract class, is a class that contains both concrete and abstract methods (methods without implementations). An abstract method must be implemented by the abstract class sub-classes. Abstract classes cannot be instantiated and need to be extended to be used.
- An interface is like a blueprint/contract of a class (or it may be thought of as a class with methods, but without their implementation). It contains empty methods that represent, what all of its subclasses should have in common. The subclasses provide the implementation for each of these methods. Interfaces are implemented.
-
What is the difference between iterator and enumeration in java?
-
Do you agree we use composition over inheritance? Composition vs Inheritance
-
Difference between method overloading and overriding.
-
Overloading happens at compile-time while Overriding happens at runtime: The binding of overloaded method call to its definition happens at compile-time however binding of overridden method call to its definition happens at runtime. More info on static vs. dynamic binding: StackOverflow.
-
Static methods can be overloaded which means a class can have more than one static method of same name. Static methods cannot be overridden, even if you declare a same static method in child class it has nothing to do with the same method of parent class as overridden static methods are chosen by the reference class and not by the class of the object.
So, for example:
public class Animal { public static void testClassMethod() { System.out.println("The static method in Animal"); } public void testInstanceMethod() { System.out.println("The instance method in Animal"); } } public class Cat extends Animal { public static void testClassMethod() { System.out.println("The static method in Cat"); } public void testInstanceMethod() { System.out.println("The instance method in Cat"); } public static void main(String[] args) { Cat myCat = new Cat(); myCat.testClassMethod(); Animal myAnimal = myCat; myAnimal.testClassMethod(); myAnimal.testInstanceMethod(); } }
Will output:
The static method in Cat // testClassMethod() is called from "Cat" reference The static method in Animal // testClassMethod() is called from "Animal" reference, // ignoring actual object inside it (Cat) The instance method in Cat // testInstanceMethod() is called from "Animal" reference, // but from "Cat" object underneath
The most basic difference is that overloading is being done in the same class while for overriding base and child classes are required. Overriding is all about giving a specific implementation to the inherited method of parent class.
Static binding is being used for overloaded methods and dynamic binding is being used for overridden/overriding methods. Performance: Overloading gives better performance compared to overriding. The reason is that the binding of overridden methods is being done at runtime.
Private and final methods can be overloaded but they cannot be overridden. It means a class can have more than one private/final methods of same name but a child class cannot override the private/final methods of their base class.
Return type of method does not matter in case of method overloading, it can be same or different. However in case of method overriding the overriding method can have more specific return type (meaning if, for example, base method returns an instance of Number class, all overriding methods can return any class that is extended from Number, but not a class that is higher in the hierarchy, like, for example, Object is in this particular case).
Argument list should be different while doing method overloading. Argument list should be same in method Overriding. It is also a good practice to annotate overridden methods with
@Override
to make the compiler be able to notify you if child is, indeed, overriding parent’s class method during compile-time.
-
-
What are the access modifiers you know? What does each one do?
- There are four access modifiers in Java language (from strictest to the most lenient):
private
variables, methods, constructors or inner classes are only visible to its' containing class and its' methods. This modifier is most commonly used, for example, to allow variable access only through getters and setters or to hide underlying implementation of classes that should not be used by user and therefore maintain encapsulation. Singleton constructor is also markedprivate
to avoid unwanted instantiation from outside.protected
can be used on variables, methods and constructors therefore allowing access only to subclasses and classes that are inside the same package as protected members' class.- Default (no keyword is used) this modifier can be applied to classes, variables, constructors and methods and allows access from classes and methods inside the same package.
public
modifier is widely-used on classes, variables, constructors and methods to grant access from any class and method anywhere. It should not be used everywhere as it implies that data marked withpublic
is not sensitive and can not be used to harm the program.
- There are four access modifiers in Java language (from strictest to the most lenient):
-
Can an Interface implement another Interface?
- Yes, an interface can implement another interface (and more than one), but it needs to use
extends
, rather thanimplements
keyword. And while you can not remove methods from parent interface, you can add new ones freely to your subinterface.
- Yes, an interface can implement another interface (and more than one), but it needs to use
-
What is Polymorphism? What about Inheritance?
-
Polymorphism in Java has two types: Compile time polymorphism (static binding) and Runtime polymorphism (dynamic binding). Method overloading is an example of static polymorphism, while method overriding is an example of dynamic polymorphism.
An important example of polymorphism is how a parent class refers to a child class object. In fact, any object that satisfies more than one IS-A relationship is polymorphic in nature.
For instance, let’s consider a class
Animal
and letCat
be a subclass ofAnimal
. So, any cat IS animal. Here, Cat satisfies the IS-A relationship for its own type as well as its super class Animal. -
Inheritance can be defined as the process where one class acquires the properties (methods and fields) of another. With the use of inheritance the information is made manageable in a hierarchical order.
The class which inherits the properties of other is known as subclass (derived class, child class) and the class whose properties are inherited is known as superclass (base class, parent class).
Inheritance uses the keyword
extends
to inherit the properties of a class. Following is the syntax of extends keyword.class Super { ..... ..... } class Sub extends Super { ..... ..... }
-
-
Multiple inheritance in Classes and Interfaces in java Blog
-
What are the design patterns? MindOrks
-
Creational patterns
-
Builder Wikipedia
-
Factory Wikipedia
-
Singleton Wikipedia A singleton is a class that can only be instantiated once. This singleton pattern restricts the instantiation of a class to one object. This is useful when exactly one object is needed to coordinate actions across the system. The concept is sometimes generalized to systems that operate more efficiently when only one object exists, or that restrict the instantiation to a certain number of objects.
-
Monostate Wikipedia
-
Fluent Interface Pattern Wikipedia
-
-
Structural patterns
-
Behavioural patterns
-
Collections and Generics
-
Arrays
vsArrayLists
. -
HashSet
vsTreeSet
. -
HashMap
vsSet
-
Stack
vsQueue
-
Explain Generics in Java?
-
Generics were included in Java language to provide stronger type checks, by allowing the programmer to define, which classes can be used with other classes
In a nutshell, generics enable types (classes and interfaces) to be parameters when defining classes, interfaces and methods. Much like the more familiar formal parameters used in method declarations, type parameters provide a way for you to re-use the same code with different inputs. The difference is that the inputs to formal parameters are values, while the inputs to type parameters are types. (Official Java Documentation)
-
This means that, for example, you can define:
List<Integer> list = new ArrayList<>();
And let the compiler take care of noticing, if you put some object, of type other than
Integer
into this list and warn you. -
It should be noted that standard class hierarchy does not apply to generic types. It means that
Integer
inList<Integer>
is not inherited from<Number>
- it is actually inherited directly from<Object>
. You can still put some constraints on what classes can be passed as a parameter into a generic by using wildcards like<?>
,<? extends MyCustomClass>
or<? super Number>
. -
While generics are very useful, late inclusion into Java language has put some restraints on their implementation - backward compatibility required them to remain just “syntactic sugar” - they are erased (type erasure) during compile-time and replaced with
object
class.
-
-
What is Java
PriorityQueue
?
Objects and Primitives
String
-
How is
String
class implemented? Why was it made immutable?-
There is no primitive variant of
String
class in Java language - all strings are just wrappers around underlying array of characters, which is declaredfinal
. This means that, once aString
object is instantiated, it cannot be changed through normal tools of the language (Reflection still can mess things up horribly, because in Java no object is truly immutable). This is whyString
variables in classes are the first candidates to be used, when you want to overridehashCode()
andequals()
of your class - you can be sure, that all their required contracts will be satisfied.Note: The String class is immutable, so that once it is created a String object cannot be changed. The String class has a number of methods, some of which will be discussed below, that appear to modify strings. Since strings are immutable, what these methods really do is create and return a new string that contains the result of the operation. (Official Java Documentation)
This class is also unique in a sense, that, when you create an instance like this:
String helloWorld = "Hello, World!";
"Hello, World!"
is called a literal and compiler creates aString
object with its' value. SoString capital = "Hello, World!".toUpperCase();
is a valid statement, that, firstly, will create an object with literal value “Hello, World!” and then will create and return another object with value “HELLO, WORLD!”
-
String
was made immutable to prevent malicious manipulation of data, when, for example, user login or other sensitive data is being send to a server.
-
-
What does it means to say that a
String
is immutable?- It means that once created,
String
object’schar[]
(its' containing value) is declaredfinal
and, therefore, it can not be changed during runtime.
- It means that once created,
-
What is
String.intern()
? When and why should it be used? -
Can you list 8 primitive types in java?
-
What is the difference between an Integer and int?
int
is a primitive data type (withboolean
,byte
,char
,short
,long
,float
anddouble
), whileInteger
(withBoolean
,Byte
,Character
,Short
,Long
,Float
andDouble
) is a wrapper class that encapsulates primitive data type, while providing useful methods to perform different tasks with it.
-
What is Autoboxing and Unboxing?
- Autoboxing and Unboxing is the process of automatic wrapping (putting in a box) and unwrapping (getting the value out) of primitive data types, that have “wrapper” classes. So
int
andInteger
can (almost always) be used interchangeably in Java language, meaning a methodvoid giveMeInt(int i) { ... }
can takeint
as well asInteger
as a parameter.
- Autoboxing and Unboxing is the process of automatic wrapping (putting in a box) and unwrapping (getting the value out) of primitive data types, that have “wrapper” classes. So
-
Typecast in Java.
- In Java, you can use casts to polymorph one class into another, compatible one. For example:
Here we see, that, while narrowing (long i = 10l; int j = (int) i; long k = j;
long i
->int j
) requires an explicit cast to make sure the programmer realizes, that there may be some data or precision loss, widening (int j
->long k
) does not require an explicit cast, because there can be no data loss (long
can take larger numbers thanint
allows).
- In Java, you can use casts to polymorph one class into another, compatible one. For example:
-
Do objects get passed by reference or value in Java? Elaborate on that.
- In Java all primitives and objects are passed by value, meaning that their copy will be manipulated in the receiving method. But there is a caveat - when you pass an object reference into a method, a copy of this reference is made, so it still points to the same object. This means, that any changes that you make to the insides of this object are retained, when the method exits.
public class Pointer { int innerField; public Pointer(int a) { this.innerField = a; } }
Will output:public class ValueAndReference { public static void main(String[] args) { Pointer a = new Pointer(0); int b = 1; print("Before:"); print("b = " + b); print("a.innerField = " + a.innerField); exampleMethod(a, b); print("After:"); print("b = " + b); print("a.innerField = " + a.innerField); } static void exampleMethod(Pointer a, int b) { a.innerField = 2; b = 10; } static void print(String text) { System.out.println(text); } }
Before: b = 1 a.innerField = 0 After: b = 1 // a new local int variable was created and operated on, so "b" didn't change a.innerField = 2 // Pointer a got its' innerField variable changed // from 0 to 2, because method was operating on // the same reference to an instance
- In Java all primitives and objects are passed by value, meaning that their copy will be manipulated in the receiving method. But there is a caveat - when you pass an object reference into a method, a copy of this reference is made, so it still points to the same object. This means, that any changes that you make to the insides of this object are retained, when the method exits.
-
What is the difference between instantiation and initialization of an object?
- Initialization is the process of the memory allocation, when a new variable is created. Variables should be explicitly given a value, otherwise they may contain a random value that remained from the previous variable that was using the same memory space. To avoid this problem, Java language assigns default (right after initialization) values to some data types:
boolean
defaults tofalse
;byte
defaults to0
;short
defaults to0
;int
defaults to0
;long
defaults to0L
;char
defaults to\u0000
;float
defaults to0.0f
;double
defaults to0.0d
;object
defaults tonull
.
- Instantiation is the process of explicitly assigning definitive value to a declared variable:
int j; // Initialized variable (int defaults to 0 right after) j = 10; // Instantiated variable
- Initialization is the process of the memory allocation, when a new variable is created. Variables should be explicitly given a value, otherwise they may contain a random value that remained from the previous variable that was using the same memory space. To avoid this problem, Java language assigns default (right after initialization) values to some data types:
-
What the difference between local, instance and class variables?
- Local variables exist only in methods that created them, they are stored separately in their respected Thread Stack (for more information, see question about Java Memory Model) and cannot have their reference passed outside of the method scope. That also means that they cannot be assigned any access modifier or made
static
- because they only exist during enclosing method’s execution and those modifiers just do not make sense, since no other outside method can get them anyway. - Instance variables are the ones, that are declared in classes and their value can be different from one instance of the class to another, but they always require that class' instance to exist.
- Class variables are those, that are marked with
static
keyword in their class' body. They can only have one value across all instances of that class (changing it in one place will change it in their class and, therefore, in all instances) and can even be retrieved without that class' instance (if their access modifier allows it).
- Local variables exist only in methods that created them, they are stored separately in their respected Thread Stack (for more information, see question about Java Memory Model) and cannot have their reference passed outside of the method scope. That also means that they cannot be assigned any access modifier or made
Java Memory Model and Garbage Collector
-
What is garbage collector? How does it work?
- All objects are allocated on the heap area managed by the JVM. As long as an object is being referenced, the JVM considers it alive. Once an object is no longer referenced and therefore is not reachable by the application code, the garbage collector removes it and reclaims the unused memory.
-
What is Java Memory Model? What contracts does it guarantee? How are its' Heap and Stack organized? Jenkov
-
What is memory leak and how does Java handle it?
-
What are strong, soft, weak and phantom references in Java?
Concurrency
-
What does the keyword
synchronized
mean? Link -
What is a
ThreadPoolExecutor
? MindOrks -
What is
volatile
modifier? Jenkov -
The clasess in the atomic package expose a common set of methods:
get
,set,
,lazyset
,compareAndSet
, andweakCompareAndSet
. Please describe them.
Exceptions
-
How does the
try{} catch{} finally{}
works? Link -
What is the difference between a
Checked Exception
and anUn-Checked Exception
?
Others
-
What is serialization? How do you implement it?
-
Serialization is the process of converting an object into a stream of bytes in order to store an object into memory, so that it can be recreated at a later time, while still keeping the object’s original state and data. In Android you may use either the Serializable, Externalizable (implements Serializable) or Parcelable interfaces.
-
While Serializable is the easiest to implement, Externalizable may be used if you need to insert custom logic into the process of serialization (although it is almost never used nowadays as it is considered a relic from early versions of Java). But it is highly recommended to use Parcelable in Android instead, as Parcelable was created exclusively for Android and it performs about 10x faster than Serializable, because Serializable uses reflection, which is a slow process and tends to create a lot of temporary objects and it may cause garbage collection to occur more often.
-
To use Serializable all you have to do is implement the interface:
/** * Implementing the Serializeable interface is all that is required */ public class User implements Serializable { private String name; private String email; public User() { } public String getName() { return name; } public void setName(final String name) { this.name = name; } public String getEmail() { return email; } public void setEmail(final String email) { this.email = email; } }
-
Parcelable requires a bit more work:
public class User implements Parcelable { private String name; private String email; /** * Interface that must be implemented and provided as a public CREATOR field * that generates instances of your Parcelable class from a Parcel. */ public static final Creator<User> CREATOR = new Creator<User>() { /** * Creates a new USer object from the Parcel. This is the reason why * the constructor that takes a Parcel is needed. */ @Override public User createFromParcel(Parcel in) { return new User(in); } /** * Create a new array of the Parcelable class. * @return an array of the Parcelable class, * with every entry initialized to null. */ @Override public User[] newArray(int size) { return new User[size]; } }; public User() { } /** * Parcel overloaded constructor required for * Parcelable implementation used in the CREATOR */ private User(Parcel in) { name = in.readString(); email = in.readString(); } public String getName() { return name; } public void setName(final String name) { this.name = name; } public String getEmail() { return email; } public void setEmail(final String email) { this.email = email; } @Override public int describeContents() { return 0; } /** * This is where the parcel is performed. */ @Override public void writeToParcel(final Parcel parcel, final int i) { parcel.writeString(name); parcel.writeString(email); } }
Note: For a full explanation of the describeContents() method see StackOverflow. In Android Studio, you can have all of the parcelable code auto generated for you, but like with everything else, it is always a good thing to try and understand everything that is happening.
-
-
What is
transient
modifier? JavaTPoint -
What are anonymous classes? OracleDoc
-
What is the difference between using
==
and.equals
on an object? GeeksForGeeks -
What is the
hashCode()
andequals()
used for? -
Why would you not call abstract method in constructor?
-
When would you make an object value
final
? -
What are these
final
,finally
andfinalize
keywords?final
is a keyword in the java language. It is used to apply restrictions on class, method and variable. Final class can’t be inherited, final method can’t be overridden and final variable value can’t be changed.class FinalExample { public static void main(String[] args) { final int x=100; x=200;//Compile Time Error because x is final } }
finally
is a code block and is used to place important code, it will be executed whether exception is handled or not.class FinallyExample { public static void main(String[] args) { try { int x=300; }catch(Exception e) { System.out.println(e); } finally { System.out.println("finally block is executed"); } } }
Finalize
is a method used to perform clean up processing just before object is garbage collected.class FinalizeExample { public void finalize() { System.out.println("finalize called"); } public static void main(String[] args) { FinalizeExample f1=new FinalizeExample(); FinalizeExample f2=new FinalizeExample(); f1=null; f2=null; System.gc(); } }
-
What does the
static
word mean in Java?- In case of
static
variable it means that this variable (its' value or the object it references) spans across all instances of enclosing class (changing it in one instance affects all others), while in case ofstatic
methods it means that these methods can be invoked without an instance of their enclosing class. It is useful, for example, when you create util classes that need not be instantiated every time you want to use them.
- In case of
-
Can a
static
method be overridden in Java?- While child class can override a static method with another static method with the same signature (return type can be downcasted), it is not truly overridden - it becomes “hidden”, but both methods can still be accessed under right circumstances (see question about overloading/overriding above).
-
When is a
static
block run?- Code inside static block is executed only once: the first time you make an object of that class or the first time you access a static member of that class (even if you never make an object of that class).
-
What is reflection? Jenkov
-
What is Dependency Injection? Can you name few libraries? Have you used any?
- Dependency injection is a very powerful technique, where you relay the task of providing object with its' dependencies on instances of other objects (OOP Composition, Wikipedia) to a separate class. This allows for fewer constructors, setters, factories and builders as all those functions are taken care of by the DI framework that you use. Also, and it may seem as a minor advantage, but if you use DI framework you need not worry about going through the project and changing all of (example names)
YourCustomInterface customInterfaceObject = new YourCustomClass();
to a new implementaion, as long as your new class (in place ofYourCustomClass
) still implementsCustomInterface
- you can just tweak the DI factory class to produce new class and voila - this new class will be automatically instantiated throughout your code. This allows for better maintenence and control over the program. Another example of DI usage is unit-testing - it allows to conveniently inject all needed dependencies and keep the amount of written code at a lower level. - One of the most popular libraries for DI for Android is Dagger 2. MindOrks
- Dependency injection is a very powerful technique, where you relay the task of providing object with its' dependencies on instances of other objects (OOP Composition, Wikipedia) to a separate class. This allows for fewer constructors, setters, factories and builders as all those functions are taken care of by the DI framework that you use. Also, and it may seem as a minor advantage, but if you use DI framework you need not worry about going through the project and changing all of (example names)
-
How is a
StringBuilder
implemented to avoid the immutable string allocation problem? -
Difference between
StringBuffer
andStringBuilder
? Link -
What’s the difference between an
Enumeration
and anIterator
? Link -
What is the difference between fail-fast and fail-safe iterators in Java?
-
What is Java NIO? Link
Core Android
Base
-
Tell all the Android application components. Android Official
-
What is the structure of an Android Application?
-
What is
Context
? How is it used? MindOrks -
What is
AndroidManifest.xml
? Android Official -
What is
Application
class?
Activity
-
What is
Activity
? MindOrks -
Explain
Activity
andFragment
lifecycle. (Complete diagram GitHub, simplified diagram for Activity, Fragment), Activity lifecycle and Fragments lifecycle -
What are “launch modes”? MindOrks
Fragments
-
What is
Fragment
? MindOrks -
What is the difference between a
Fragment
and anActivity
? Explain the relationship between the two. -
Why is it recommended to use only the default constructor to create a
Fragment
? StackOverflow -
How would you communicate between two Fragments? Android Official
-
What is retained
Fragment
? AndroidDesignPatterns
Views and ViewGroups
-
What is
View
in Android? MindOrks -
Difference between
View.GONE
andView.INVISIBLE
? StackOverflow -
Can you create custom views? How? MindOrks
-
What are ViewGroups and how they are different from the Views?
-
What is a canvas?
-
What is a
SurfaceView
? -
Relative Layout vs Linear Layout. MindOrks
-
Tell about Constraint Layout MindOrks
-
Do you know what is the view tree? How can you optimize its depth?
Displaying Lists of Content
-
What is the difference between
ListView
andRecyclerView
? -
What is the ViewHolder pattern? Why should we use it?
-
What is
SnapHelper
? MindOrks
Dialogs and Toasts
-
What is
Dialog
in Android?Dialog -
What is
Toast
in Android?Toast -
What the difference between
Dialog
andDialog Fragment
?
Intents and Broadcasting
-
What is
Intent
? StackOverflow -
What is an Implicit
Intent
? StackOverflow -
What is an Explicit
Intent
? -
What is a
BroadcastReceiver
? StackOverflow -
What is a
LocalBroadcastManager
? Developer Android -
What is the function of an
IntentFilter
? StackOverflow -
What is a Sticky
Intent
? AndroidInterview -
Describe how broadcasts and intents work to be able to pass messages around your app?
-
What is a
PendingIntent
? -
What are the different types of Broadcasts?
Services
-
What is
Serivce
? Developer Android -
Service
vsIntentService
. MindOrks -
What is a
JobScheduler
? Vogella
Inter-process Communication
-
How can two distinct Android apps interact? Developer Android
-
Is it possible to run an Android app in multiple processes? How?
-
What is AIDL? Enumerate the steps in creating a bounded service through AIDL. Developer Android
-
What can you use for background processing in Android? Developer Android
-
What is a
ContentProvider
and what is it typically used for? Developer Android Developer Android
Long-running Operations
-
How would you perform a long-running operation in an application?
-
Why should you avoid to run non-ui code on the main thread?
-
What is ANR? How can the ANR be prevented? Developer Android
-
What is an
AsyncTask
? Developer Android -
What are the problems in asynctask?
-
When would you use java thread instead of an asynctask?
-
What is a
Loader
? (Deprecated) Developer Android -
What is the relationship between the life cycle of an
AsyncTask
and anActivity
? What problems can this result in? How can these problems be avoided? -
Explain
Looper
,Handler
andHandlerThread
. MindOrks and MindOrks Video
Working With Multimedia Content
-
How do you handle bitmaps in Android as it takes too much memory? Developer Android Developer Android
-
What is the difference between a regular
Bitmap
and a nine-patch image? -
Tell about the
Bitmap
pool. MindOrks -
How to play sounds in Android? Vogella
Data Saving
-
How to persist data in an Android app? MindOrks
-
What is ORM? How does it work?
-
How would you preserve
Activity
state during a screen rotation? StackOverflow -
What are different ways to store data in your Android app? Developer Android
Look and Feel
-
What is a
Spannable
? Medium -
What is a
SpannableString
?- A SpannableString has immutable text, but its span information is mutable. Use a SpannableString when your text doesn’t need to be changed but the styling does. Spans are ranges over the text that include styling information like color, highlighting, italics, links, etc
Memory Optimizations
-
What is the
onTrimMemory()
method?- Called when the operating system has determined that it is a good time for a process to trim unneeded memory from its process. This will happen for example when it goes in the background and there is not enough memory to keep as many background processes running as desired
-
How does the OutOfMemory happens?
- Thrown when the Java Virtual Machine cannot allocate an object because it is out of memory, and no more memory could be made available by the garbage collector
Memory Optimizations
-
What is the
onTrimMemory()
method? Developer Android -
How does the OutOfMemory happens? Geeksforgeeks
-
How do you find memory leaks in Android applications? Practical Guide - MindOrks MindOrks
Battery Life Optimizations
-
How to reduce battery usage in an android application? MindOrks
-
What is Doze? What about App Standby? Developer Android
-
What is
overdraw
? Developer Android
Supporting Different Screen Sizes
- How did you support different types of resolutions?
Permissions
- What are the different protection levels in permission?
Native Programming
Android System Internal
-
What is the Dalvik Virtual Machine?
-
What is the difference JVM, DVM and ART?
-
What are the differences between Dalvik and ART?
-
What is DEX?
-
Can you manually call the Garbage collector?
Debugging and Programming Tools
-
What is ADB?
-
What is DDMS and what can you do with it?
-
What is the StrictMode? MindOrks
-
What is Lint? What is it used for? MindOrks
Others
-
OkHttp Interceptors. MindOrks
-
Why Bundle class is used for data passing and why cannot we use simple Map data structure
-
How do you troubleshoot a crashing application?
-
Explain Android notification system?
-
What is the difference between Serializable and Parcelable? Which is the best approach in Android?
-
Have you developed widgets? Describe. MindOrks
-
What is AAPT?
-
What is the best way to update the screen periodically?
-
FlatBuffers vs JSON. MindOrks
-
HashMap
,ArrayMap
andSparseArray
MindOrks -
How to handle multi-touch in android GitHub
-
How to implement XML namespaces?
-
What is the support library? Why was it introduced?MartianCraft
-
What is Android Data Binding? Developer Android
-
What are Android Architecture Components? MindOrks
-
How to implement search using RxJava operators? MindOrks
Architecture
-
Describe the architecture of your last app.
-
Describe MVP. MindOrks
-
MVC vs MVP vs MVVM architecture. MindOrks
-
What is presenter?
-
What is model?
-
Describe MVC.
-
Describe MVI
-
Describe the repository pattern
-
What is controller?
-
Describe MVVM. GitHub
-
Tell something about clean code MindOrks
Design Problem
-
Design Uber App.
-
Design Facebook App.
-
Design Facebook Near-By Friends App.
-
Design WhatsApp.
-
Design SnapChat.
-
Design problems based on location based app.
Tools And Technologies
-
Git. MindOrks Youtube
-
RxJava. MindOrks
-
Dagger 2. MindOrks
-
Android Development Useful Tools. MindOrks
-
Firebase. Firebase.google.com
Android Test Driven Development
-
What is Espresso? Developer Android
-
What is Robolectric? Robolectric
-
What are the disadvantages of using Roboelectric?
-
What is UI-Automator? Developer Android
-
Explain unit test. Unit Test
-
Explain instrumented test.
-
Have you done unit testing or automatic testing?
-
Why Mockito is used? Official site
-
Describe JUnit test.
Others
-
What is Android Jetpack? MindOrks
-
Describe how REST APIs work. What is REST?
-
Describe other forms of web API architecutre. [GraphQL] (https://medium.com/mindorks/what-is-graphql-and-using-it-on-android-ab8e493abdd7) [SOAP] (https://www.w3.org/TR/ws-arch/)
-
Describe SQLite. MindOrks
-
Describe database.
-
Project Management tool - trello, basecamp, kanban, jira, asana.
-
About build System - gradle, maven, ant, buck.
-
About multiple apk for android application. MindOrks
-
Reverse Engineering an APK.
-
What is proguard used for? MindOrks
-
What is obfuscation? What is it used for? What about minification?
-
How do you build your apps for release?
-
How do you control the application version update to specific number of users?
-
Can we identify users who have uninstalled our application?
-
Implement Search Using RxJava Operators. MindOrks
-
APK Size Reduction. MindOrks
-
Android Development Best Practices. MindOrks
-
Android Code Style And Guidelines. MindOrks
-
Have you tried Kotlin? MindOrks
-
What are Coroutines in Kotlin? MindOrks
-
What are the metrics that you should measure continuously while android application development? MindOrks
-
What is Chrome Custom Tabs? How to display web content in your app? MindOrks
Found this project useful :heart:
- Support by clicking the :star: button on the upper right of this page. :v:
Check out MindOrks awesome open source projects here
License
Copyright (C) 2017 MINDORKS NEXTGEN PRIVATE LIMITED
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
Contributing to Android Interview Questions
Just make pull request. You are in!