oatpp/oatpp
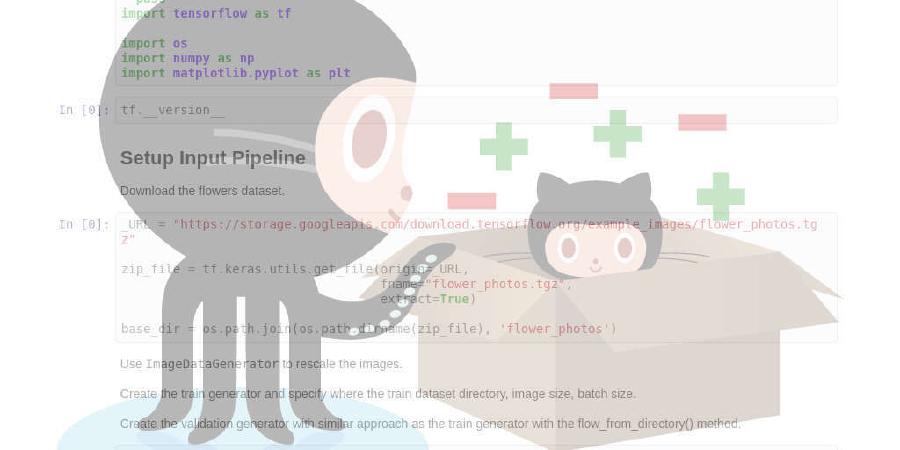
Modern Web Framework for C++. High performance, simple API, cross platform, zero dependency.
repo name | oatpp/oatpp |
repo link | https://github.com/oatpp/oatpp |
homepage | https://oatpp.io/ |
language | C++ |
size (curr.) | 2154 kB |
stars (curr.) | 2242 |
created | 2018-03-13 |
license | Apache License 2.0 |
Oat++

Oat++ is the modern Web Framework for C++. It’s fully loaded and contains all necessary components for effective production level development. It’s also light and has small memory footprint.
- Website
- Docs
- Api Reference
- Supported Platforms
- Latest Benchmarks: 5 Million WebSockets
Contributors wanted!
Join the community
- Join discussion on Gitter. oat++ framework/Lobby
- Follow us on Twitter for latest news. @oatpp_io
- Join community on Reddit. r/oatpp
High Level Overview
- API Controller And Request Mapping
- Swagger-UI Annotations
- API Client - Retrofit / Feign Like Client
- Object Mapping
API Controller And Request Mapping
For more info see Api Controller
Declare Endpoint
ENDPOINT("PUT", "/users/{userId}", putUser,
PATH(Int64, userId),
BODY_DTO(dto::UserDto::ObjectWrapper, userDto))
{
userDto->id = userId;
return createDtoResponse(Status::CODE_200, m_database->updateUser(userDto));
}
Add CORS for Endpoint
For more info see Api Controller / CORS
ADD_CORS(putUser)
ENDPOINT("PUT", "/users/{userId}", putUser,
PATH(Int64, userId),
BODY_DTO(dto::UserDto::ObjectWrapper, userDto))
{
userDto->id = userId;
return createDtoResponse(Status::CODE_200, m_database->updateUser(userDto));
}
Endpoint with Authorization
For more info see Api Controller / Authorization
using namespace oatpp::web::server::handler;
ENDPOINT("PUT", "/users/{userId}", putUser,
AUTHORIZATION(std::shared_ptr<DefaultBasicAuthorizationObject>, authObject),
PATH(Int64, userId),
BODY_DTO(dto::UserDto::ObjectWrapper, userDto))
{
OATPP_ASSERT_HTTP(authObject->userId == "Ivan" && authObject->password == "admin", Status::CODE_401, "Unauthorized");
userDto->id = userId;
return createDtoResponse(Status::CODE_200, m_database->updateUser(userDto));
}
Swagger-UI Annotations
For more info see Endpoint Annotation And API Documentation
Additional Endpoint Info
ENDPOINT_INFO(putUser) {
// general
info->summary = "Update User by userId";
info->addConsumes<dto::UserDto::ObjectWrapper>("application/json");
info->addResponse<dto::UserDto::ObjectWrapper>(Status::CODE_200, "application/json");
info->addResponse<String>(Status::CODE_404, "text/plain");
// params specific
info->pathParams["userId"].description = "User Identifier";
}
ENDPOINT("PUT", "/users/{userId}", putUser,
PATH(Int64, userId),
BODY_DTO(dto::UserDto::ObjectWrapper, userDto))
{
userDto->id = userId;
return createDtoResponse(Status::CODE_200, m_database->updateUser(userDto));
}
API Client - Retrofit / Feign Like Client
For more info see Api Client
Declare Client
class UserService : public oatpp::web::client::ApiClient {
public:
API_CLIENT_INIT(UserService)
API_CALL("GET", "/users", getUsers)
API_CALL("GET", "/users/{userId}", getUserById, PATH(Int64, userId))
};
Using API Client
auto response = userService->getUserById(id);
auto user = response->readBodyToDto<dto::UserDto>(objectMapper);
Object Mapping
For more info see Data Transfer Object (DTO).
Declare DTO
class UserDto : public oatpp::data::mapping::type::Object {
DTO_INIT(UserDto, Object)
DTO_FIELD(Int64, id);
DTO_FIELD(String, name);
};
Serialize DTO Using ObjectMapper
using namespace oatpp::parser::json::mapping;
auto user = UserDto::createShared();
user->id = 1;
user->name = "Ivan";
auto objectMapper = ObjectMapper::createShared();
auto json = objectMapper->writeToString(user);
Read Next
Examples:
- Media-Stream (Http-Live-Streaming) - Example project of how-to build HLS-streaming server using oat++ Async-API.
- CRUD - Example project of how-to create basic CRUD endpoints.
- AsyncApi - Example project of how-to use asynchronous API for handling large number of simultaneous connections.
- ApiClient-Demo - Example project of how-to use Retrofit-like client wrapper (ApiClient) and how it works.
- TLS-Libressl - Example project of how-to setup secure connection and serve via HTTPS.
- Consul - Example project of how-to use oatpp::consul::Client. Integration with Consul.
- PostgreSQL - Example of a production grade entity service storing information in PostgreSQL. With Swagger-UI and configuration profiles.
- WebSocket - Collection of oatpp WebSocket examples.