typicode/lowdb
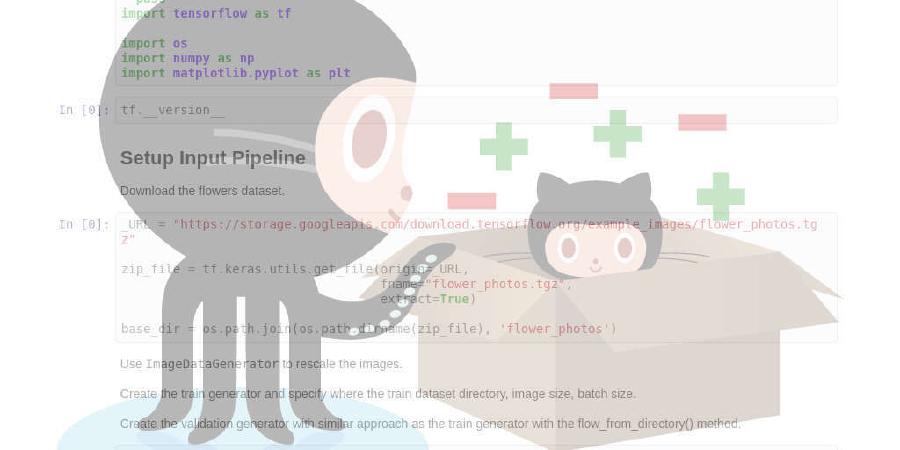
lowdb is a small local JSON database powered by Lodash (supports Node, Electron and the browser)
repo name | typicode/lowdb |
repo link | https://github.com/typicode/lowdb |
homepage | |
language | JavaScript |
size (curr.) | 1263 kB |
stars (curr.) | 12467 |
created | 2014-04-02 |
license | MIT License |
Lowdb
Small JSON database for Node, Electron and the browser. Powered by Lodash. :zap:
db.get('posts')
.push({ id: 1, title: 'lowdb is awesome'})
.write()
Usage
npm install lowdb
const low = require('lowdb')
const FileSync = require('lowdb/adapters/FileSync')
const adapter = new FileSync('db.json')
const db = low(adapter)
// Set some defaults (required if your JSON file is empty)
db.defaults({ posts: [], user: {}, count: 0 })
.write()
// Add a post
db.get('posts')
.push({ id: 1, title: 'lowdb is awesome'})
.write()
// Set a user using Lodash shorthand syntax
db.set('user.name', 'typicode')
.write()
// Increment count
db.update('count', n => n + 1)
.write()
Data is saved to db.json
{
"posts": [
{ "id": 1, "title": "lowdb is awesome"}
],
"user": {
"name": "typicode"
},
"count": 1
}
You can use any of the powerful lodash functions, like _.get
and _.find
with shorthand syntax.
// For performance, use .value() instead of .write() if you're only reading from db
db.get('posts')
.find({ id: 1 })
.value()
Lowdb is perfect for CLIs, small servers, Electron apps and npm packages in general.
It supports Node, the browser and uses lodash API, so it’s very simple to learn. Actually, if you know Lodash, you already know how to use lowdb :wink:
Important lowdb doesn’t support Cluster and may have issues with very large JSON files (~200MB).
Install
npm install lowdb
Alternatively, if you’re using yarn
yarn add lowdb
A UMD build is also available on unpkg for testing and quick prototyping:
<script src="https://unpkg.com/lodash@4/lodash.min.js"></script>
<script src="https://unpkg.com/lowdb@0.17/dist/low.min.js"></script>
<script src="https://unpkg.com/lowdb@0.17/dist/LocalStorage.min.js"></script>
<script>
var adapter = new LocalStorage('db')
var db = low(adapter)
</script>
API
low(adapter)
Returns a lodash chain with additional properties and functions described below.
db.[…].write() and db.[…].value()
write()
writes database to state.
On the other hand, value()
is just _.prototype.value() and should be used to execute a chain that doesn’t change database state.
db.set('user.name', 'typicode')
.write()
Please note that db.[...].write()
is syntactic sugar and equivalent to
db.set('user.name', 'typicode')
.value()
db.write()
db._
Database lodash instance. Use it to add your own utility functions or third-party mixins like underscore-contrib or lodash-id.
db._.mixin({
second: function(array) {
return array[1]
}
})
db.get('posts')
.second()
.value()
db.getState()
Returns database state.
db.getState() // { posts: [ ... ] }
db.setState(newState)
Replaces database state.
const newState = {}
db.setState(newState)
db.write()
Persists database using adapter.write
(depending on the adapter, may return a promise).
// With lowdb/adapters/FileSync
db.write()
console.log('State has been saved')
// With lowdb/adapters/FileAsync
db.write()
.then(() => console.log('State has been saved'))
db.read()
Reads source using storage.read
option (depending on the adapter, may return a promise).
// With lowdb/FileSync
db.read()
console.log('State has been updated')
// With lowdb/FileAsync
db.read()
.then(() => console.log('State has been updated'))
Adapters API
Please note this only applies to adapters bundled with Lowdb. Third-party adapters may have different options.
For convenience, FileSync
, FileAsync
and LocalBrowser
accept the following options:
- defaultValue if file doesn’t exist, this value will be used to set the initial state (default:
{}
) - serialize/deserialize functions used before writing and after reading (default:
JSON.stringify
andJSON.parse
)
const adapter = new FileSync('array.yaml', {
defaultValue: [],
serialize: (array) => toYamlString(array),
deserialize: (string) => fromYamlString(string)
})
Guide
How to query
With lowdb, you get access to the entire lodash API, so there are many ways to query and manipulate data. Here are a few examples to get you started.
Please note that data is returned by reference, this means that modifications to returned objects may change the database. To avoid such behaviour, you need to use .cloneDeep()
.
Also, the execution of methods is lazy, that is, execution is deferred until .value()
or .write()
is called.
Reading from existing JSON file
If you are reading from a file adapter, the path is relative to execution path (CWD) and not to your code.
my_project/
src/
my_example.js
db.json
So then you read it like this:
// file src/my_example.js
const adapter = new FileSync('db.json')
// With lowdb/FileAsync
db.read()
.then(() => console.log('Content of my_project/db.json is loaded'))
Examples
Check if posts exists.
db.has('posts')
.value()
Set posts.
db.set('posts', [])
.write()
Sort the top five posts.
db.get('posts')
.filter({published: true})
.sortBy('views')
.take(5)
.value()
Get post titles.
db.get('posts')
.map('title')
.value()
Get the number of posts.
db.get('posts')
.size()
.value()
Get the title of first post using a path.
db.get('posts[0].title')
.value()
Update a post.
db.get('posts')
.find({ title: 'low!' })
.assign({ title: 'hi!'})
.write()
Remove posts.
db.get('posts')
.remove({ title: 'low!' })
.write()
Remove a property.
db.unset('user.name')
.write()
Make a deep clone of posts.
db.get('posts')
.cloneDeep()
.value()
How to use id based resources
Being able to get data using an id can be quite useful, particularly in servers. To add id-based resources support to lowdb, you have 2 options.
shortid is more minimalist and returns a unique id that you can use when creating resources.
const shortid = require('shortid')
const postId = db
.get('posts')
.push({ id: shortid.generate(), title: 'low!' })
.write()
.id
const post = db
.get('posts')
.find({ id: postId })
.value()
lodash-id provides a set of helpers for creating and manipulating id-based resources.
const lodashId = require('lodash-id')
const FileSync = require('lowdb/adapters/FileSync')
const adapter = new FileSync('db.json')
const db = low(adapter)
db._.mixin(lodashId)
// We need to set some default values, if the collection does not exist yet
// We also can store our collection
const collection = db
.defaults({ posts: [] })
.get('posts')
// Insert a new post...
const newPost = collection
.insert({ title: 'low!' })
.write()
// ...and retrieve it using its id
const post = collection
.getById(newPost.id)
.value()
How to create custom adapters
low()
accepts custom Adapter, so you can virtually save your data to any storage using any format.
class MyStorage {
constructor() {
// ...
}
read() {
// Should return data (object or array) or a Promise
}
write(data) {
// Should return nothing or a Promise
}
}
const adapter = new MyStorage(args)
const db = low(adapter)
See src/adapters for examples.
How to encrypt data
FileSync
, FileAsync
and LocalStorage
accept custom serialize
and deserialize
functions. You can use them to add encryption logic.
const adapter = new FileSync('db.json', {
serialize: (data) => encrypt(JSON.stringify(data)),
deserialize: (data) => JSON.parse(decrypt(data))
})
Changelog
See changes for each version in the release notes.
Limits
Lowdb is a convenient method for storing data without setting up a database server. It is fast enough and safe to be used as an embedded database.
However, if you seek high performance and scalability more than simplicity, you should probably stick to traditional databases like MongoDB.
License
MIT - Typicode :cactus: